- WebPack Backstage is a WordPress Speed Optimization & Security Service that keeps your website running at top speeds by fixing all of the hidden technical problems that are gumming up the works. Poor security can cause a lot of problems for your WordPress website. It leaves all of your data vulnerable to exploitation by hackers.
- Webpack Config for Wordpress This Repo is suited as Starter-Template for Blanc Wordpress Themes. Webpack is used to compile Sass and CSS is then given to Project folder. With Browsersync, a refresh of a started Wordpress Server is triggered.
- Webpack Makes It to the WordPress Core as A JS Bundle Manager In late 2014, to split JavaScript files into maintainable modules, Browserify was introduced. Recently, WordPress is rapidly moving towards JavaScript. A significant part of Gutenberg is composed of JavaScript.
The idea of using Webpack as a bundler has been on my mind since launching this site. Originally I was relying on code minification plugins in WordPress, but began to see some unpredictable behavior (plus I was dying for the ability to use SASS to style my site). Incorporating Webpack gave me more control, the ability to work with SASS, and laid the groundwork for exploring code chunking in the future.
Webpack is a module bundler. Its main purpose is to bundle JavaScript files for usage in a browser, yet it is also capable of transforming, bundling, or packaging just about any resource or asset.
Installing and Initializing Webpack
I started in the root folder of my WordPress Theme (for me this was [WordPress-root]/wp-content/themes/taylor/). Once inside, I initialized a new Node project:
Then I installed Webpack and the Webpack CLI:
With npm’s auto-generated package.json file, I added three script options as shorthand for the Webpack build and watch commands. I differentiated between a development build and a production build because setting the build mode to “production” provides extra optimization inside of Webpack1:
Organizing the Source Code
How to organize code is absolutely a matter of preference, and I don’t think there is really any wrong way to do it as long as things feel clean and organized. For this guide, I created a “js” directory and a “css”directory inside of my theme’s root folder. Each of these directories had a “src” folder, which contained my source code, and a “build” folder, which acted as the build target for my Webpack compiled assets:
Configuring the JavaScript Build (with Babel and Minification)
I used a pretty standard JavaScript build configuration, and decided to incorporate Babel for maximum browser compatibility. Code minification was also plug-and-play using the uglifyjs-webpack-plugin.
To install the Babel and the code minification dependencies, I ran:
Next, I created the Webpack config file – webpack.config.js – in my theme’s root folder with the following contents:
Configuring the SASS Build
Incorporating SASS compilation involved more trial and error, especially with some of the new Webpack 4 configurations. Webpack 4 introduced the mini-css-extract-plugin, which replaces the ever-popular extract-text-webpack-plugin2. Essentially this plugin allows Webpack to extract CSS code into its own individual file separate from the rest of the JavaScript code. In my build, I also included the optimize-css-assets-webpack-plugin to minify the compiled CSS:
After installing dependences, it was time to make the following updates to my webpack.config.js file:
- Including the mini-css-extract-plugin and optimize-css-assets-webpack-plugin for use in our config
- Adding ./css/src/main.scss as an entry point
- Defining a new module rule to handle .sass and .scss files with the mini-css-extract-plugin and sass-loader
- Specifying the output file name in the plugins section
- Adding the optimize-css-assets-webpack-plugin as an additional minimizer
Here is the updated webpack.config.js file that contains both the JavaScript and SASS configurations:
Including the Gutenberg Block Editor styles in the SASS build (Optional)
Indesign cc 2020. WordPress 5+ ships with Gutenberg, the new and improved Block Editor used for authoring content. The CSS styles that support the Block Editor are served automatically by WordPress as a separate enqueued script.
In order to bundle these styles into the Webpack SASS build, I had to first disable the enqueued script in my functions.php:
Next I had to modify my main app.scss SASS build entry point (./taylor/css/src/app.scss) to add an include referencing the Block Editor styles from within wp-includes:
Determining the relative path was tricky, but trying the ‘cd’ command from the same working directory as my main.scss file helped me figure out how many ./s to include.
Loading the compiled assets into WordPress
Now that Webpack was compiling the JavaScript and CSS into their respective build directories, the last step was to enqueue these assets into my WordPress theme. This required a few lines in my theme’s functions.php:
Source Control and .gitignore
In order to avoid adding build files and node_modules to my git repository, I added the following lines to my .gitignore file:
Versioning the JavaScript and CSS Assets
Given that my WordPress instance was sitting behind an AWS CloudFront caching layer, it made make sense to version the Webpack compiled JavaScript and CSS assets so that each version could be accessed (and cached) independently. To do this, I simply added a hash to the output filenames in my webpack.config.js file:
Next, I incorporated the clean-webpack-plugin to remove old JavaScript and CSS files from my build directories (which made it easier to find the compiled JavaScript and CSS files when enqueuing them in WordPress):
Note: newer versions of CleanWebpackPlugin expect a full object as the configuration parameter (Thank you Moochou for pointing this out below!). For example:
Now that Webpack was producing files with unique and versioned filenames, I needed a way to enqueue them in WordPress without knowing the hash value in their filenames. I ended up using the following logic in order to do this:
- Webpack4 Release Nodes
- The Future of [extract-text-webpack-plugin]
Asset bundlers are an important part of any modern front-end workflow. With the ubiquity of CSS pre-processors and JS-centric apps, UX developers need a simple but customizable solution for compiling, minifying, linting, and optimizing bundled source files. Past alternatives for most people probably included GUI apps like CodeKit or task runners like Grunt or Gulp. Since 2016 however, Webpack has emerged as one of the most powerful, extensible and popular asset bundlers.
Webpack comes with features like minification and code splitting out of the box. This makes it an appealing choice for WordPress theme developers who want to keep their enqueued assets lean. This tutorial will walk you through the basics of how to add webpack to your next theme and customize the config. (To just view a diff of the changes outlined here, check out this pull request.)
1. Start with a copy of Underscores
For this tutorial we’re going to use a copy of underscores, a bare-bones starter theme from Automattic. You can clone underscores from the Automattic GitHub repo and do a find & replace to update the project namespaces, or you can download a copy from underscores.me, and select the “_sassify!” option behind the “Advanced Options” toggle.
2. Prerequisites: Node.js & NPM
Ensure you have up to date versions of Node.js and npm installed. Most front-end developers probably took care of this when they set up their dev environment, but for those of you who are new to the command line, you’ll want to download an installer here. If you’ve completed this step successfully, you should see results similar to these in your terminal:
3. Add a package.json file
To work with npm and keep your project dependencies organized, you’ll want to add a package.json
file in the root directory of your project. You can run npm init
and follow the prompts, use the boilerplate generated by npm init -y
, or just copy this basic example of a filled out starter package:

Note: When using this sample, be sure to update your project names and GitHub repo links.
We’ll also want to take this opportunity to add a Git ignore file, since we’ll be adding lots (hundreds) of node modules to the project which we don’t want to track. Just create a .gitignore
dotfile in your project root, or if you have one already, be sure it includes these lines:
4. Install Webpack as Project Dependency
You can install webpack via the command line using npm, with the following command:
This is the shorthand equivalent of npm install webpack --save-dev
which will install the latest version of webpack via npm, and then saves it to our list of dev dependencies in our package.json
file.
In order to work with webpack on the command line, you’ll also need to install the webpack cli tools:
Webpack Wordpress Tutorial

At this point you should be able to run the command npx webpack -v
(yes that’s npx) and immediately see a webpack version returned. If you run this command and see a progress bar or a prompt asking you to install webpack, it wasn’t added correctly.
5. Add A Basic Webpack Config
Add a basic webpack config, defining an entry point and and an output pattern.
Our entry point in this case will be named site
and will point to an index.js
file in ./src/site/
. This syntax allows us to define scalable configurations with multiple entry points that compile unique site components (for example, one per WordPress template type) as individual bundles that can be enqueued separately.
With the multiple entry points syntax, we want to define an output filename using output patterns to ensure each bundle is unique, and in this case references the entry point name that generated it.
We’ll also need to add the entry point file itself, which for now can just be some placeholder content.
With the config and entry point files in place, we’re ready to compile our project assets with webpack. You can test if your new webpack config file works with this command that compiles a production build:
If that works, we can add the build and watch commands to our package.json
file under scripts, with the following commands:
Wordpress Upload Plugin
Now we can run a production build using just npm run build
at the command line, or npm run start
to run a watch build that recompiles unminified bundles whenever changes are detected.

Webpack Wordpress Plugin
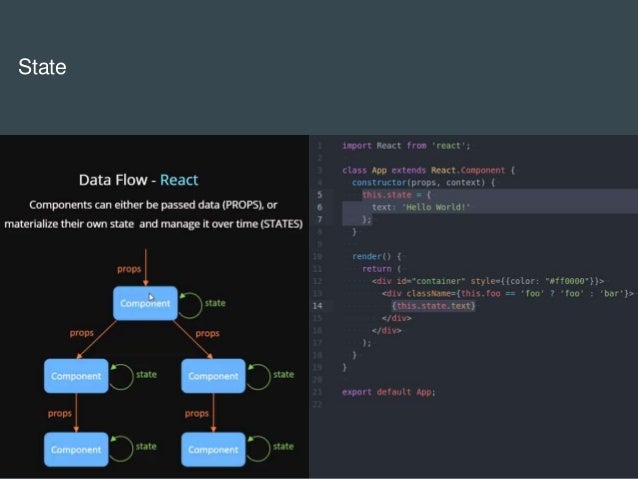
Wrapping Up
Webpack Wordpress Jquery
This tutorial covered the basics of how to add webpack as a project dependency and set up a basic custom config file. For a simple diff view of the changes outlined here, take a look at this pull request. Keep a look out for Part 2: Loading your theme files into Webpack and compiling them.
